{ "cells": [ { "cell_type": "markdown", "metadata": {}, "source": [ "# COVID-19 Interactive Analysis Dashboard" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## What is COVID-19?\n", "\n", "> Coronaviruses are a large family of viruses that may cause respiratory illnesses in humans ranging from common colds to more severe conditions such as Severe Acute Respiratory Syndrome (SARS) and Middle Eastern Respiratory Syndrome (MERS).1\n", "'Novel coronavirus' is a new, previously unidentified strain of coronavirus. The novel coronavirus involved in the current outbreak has been named SARS-CoV-2 by the World Health Organization (WHO). 3The disease it causes has been named “coronavirus disease 2019” (or “COVID-19”).`\n", "\n", "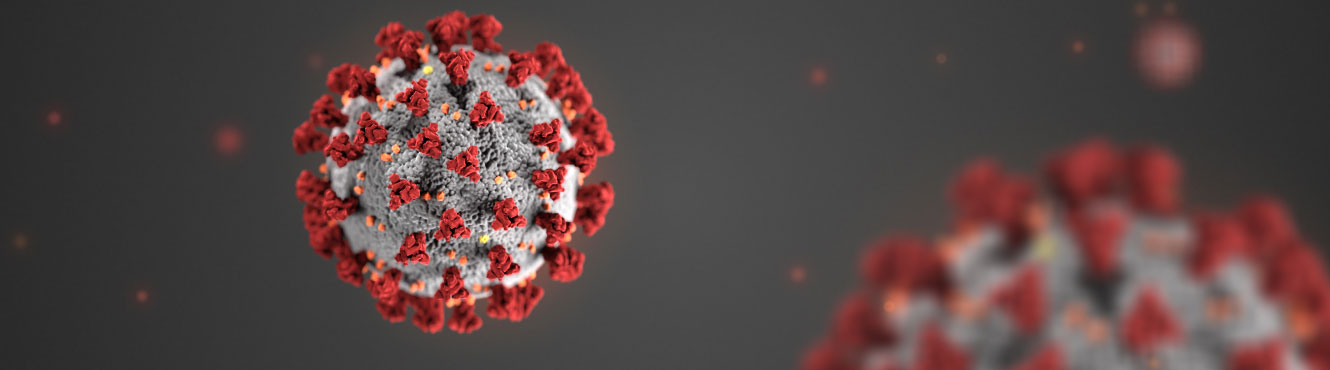" ] }, { "cell_type": "code", "execution_count": 1, "metadata": {}, "outputs": [], "source": [ "# importing libraries\n", "\n", "from __future__ import print_function\n", "from ipywidgets import interact, interactive, fixed, interact_manual\n", "from IPython.core.display import display, HTML\n", "\n", "import numpy as np\n", "import pandas as pd\n", "import matplotlib.pyplot as plt\n", "import plotly.express as px\n", "#import folium\n", "import plotly.graph_objects as go\n", "import seaborn as sns\n", "import ipywidgets as widgets" ] }, { "cell_type": "code", "execution_count": 2, "metadata": {}, "outputs": [], "source": [ "# loading data right from the source:\n", "death_df = pd.read_csv('https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_covid19_deaths_global.csv')\n", "confirmed_df = pd.read_csv('https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_covid19_confirmed_global.csv')\n", "recovered_df = pd.read_csv('https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_covid19_recovered_global.csv')\n", "country_df = pd.read_csv('https://raw.githubusercontent.com/CSSEGISandData/COVID-19/web-data/data/cases_country.csv')" ] }, { "cell_type": "code", "execution_count": 3, "metadata": {}, "outputs": [], "source": [ "# confirmed_df.head()" ] }, { "cell_type": "code", "execution_count": 4, "metadata": {}, "outputs": [], "source": [ "# recovered_df.head()" ] }, { "cell_type": "code", "execution_count": 5, "metadata": {}, "outputs": [], "source": [ "# death_df.head()" ] }, { "cell_type": "code", "execution_count": 6, "metadata": {}, "outputs": [], "source": [ "# country_df.head()" ] }, { "cell_type": "code", "execution_count": 7, "metadata": {}, "outputs": [], "source": [ "# data cleaning\n", "\n", "# renaming the df column names to lowercase\n", "country_df.columns = map(str.lower, country_df.columns)\n", "confirmed_df.columns = map(str.lower, confirmed_df.columns)\n", "death_df.columns = map(str.lower, death_df.columns)\n", "recovered_df.columns = map(str.lower, recovered_df.columns)\n", "\n", "# changing province/state to state and country/region to country\n", "confirmed_df = confirmed_df.rename(columns={'province/state': 'state', 'country/region': 'country'})\n", "recovered_df = confirmed_df.rename(columns={'province/state': 'state', 'country/region': 'country'})\n", "death_df = death_df.rename(columns={'province/state': 'state', 'country/region': 'country'})\n", "country_df = country_df.rename(columns={'country_region': 'country'})\n", "# country_df.head()" ] }, { "cell_type": "code", "execution_count": 8, "metadata": {}, "outputs": [], "source": [ "# total number of confirmed, death and recovered cases\n", "confirmed_total = int(country_df['confirmed'].sum())\n", "deaths_total = int(country_df['deaths'].sum())\n", "recovered_total = int(country_df['recovered'].sum())\n", "active_total = int(country_df['active'].sum())" ] }, { "cell_type": "code", "execution_count": 9, "metadata": {}, "outputs": [ { "data": { "text/html": [ "